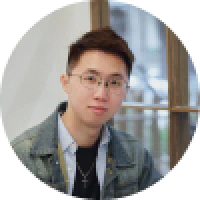
Build a Form Helper capable Form Object in Rails
The Form Object is a common pattern in Rails when our form becomes complex. But the tutorial in network’s example usually incapable of Rails’ form helper.
And I start thinking about is it possible to support form helpers without too many changes?
Common Form Object Implementation
To improve our form object, we have to know about the original version we are using.
1class RegistrationForm
2 include ActiveModel::Model
3 include ActiveModel::Validations
4
5 attr_accessor :email, :password, :password_confirmation
6
7 def initialize(user, params = {})
8 @user = user
9 super params
10 end
11
12 # ...
13
14 def attributes
15 {
16 email: @email,
17 password: @password
18 }
19 end
20
21 def save
22 return unless valid?
23
24 @user.assign_attributes(attributes)
25 @user.save
26 end
27end
This is a common form object you can find in the network, it gives us model-like behavior which can use it in the controller without too many changes.
But in the view, our form helper has to set method and URL at any time.
1<%= form_for @form, method: :post, url: users_path do |f| %>
2<% # ... %>
3<% end %>
The Form Helper
To improve the form object, I starting review the source code of form helper.
In the action_view/helpers/form_helper.rb#L440, the ActiveView will try to apply_form_for_options!
if we give a object for it.
1apply_form_for_options!(record, object, options)
In the apply_form_for_options!
method, we can find it set the method
and url
.
1action, method = object.respond_to?(:persisted?) && object.persisted? ? [:edit, :patch] : [:new, :post]
2# ...
3options[:url] ||= if options.key?(:format)
4 polymorphic_path(record, format: options.delete(:format))
5else
6 polymorphic_path(record, {})
7end
That means if our form object can provide the same interface to the form helper, it will correctly configure the form’s method and URL without extra work.
The persisted?
When the form helper decides to use POST
to create a new object or use PUT
to update an existing object. It depends on the model’s persisted?
method.
That means we can add persisted?
method to our form object to make form helper can detect it.
1class BaseForm
2 # ...
3
4 def initialize(record, params = {})
5 @record = record
6 super params
7 end
8
9 def persisted?
10 @record && @record.persisted?
11 end
12end
But there has another better way to implement it, we can use delegate
which provided by ActiveSupport.
1class BaseForm
2 # ...
3
4 delegate :persisted?, to: :@record, allow_nil: true
5
6 def initialize(record, params = {})
7 @record = record
8 super params
9 end
10end
The to_param and model_name
The URL is generated by polymorphic_path
and it uses model_name
and to_param
to generate the path.
We can try it in the Rails console:
1> app.polymorphic_path(User.new)
2=> "/users"
3> app.polymorphic_path(User.last)
4=> "/users/1234"
And when we add delegate model_name
and to_param
to our form object, we can get the same result.
1delegate :persisted?, :model_name, :to_param, to: :@record, allow_nil: true
And check it again:
1> app.polymorphic_path(RegistrationForm.new(User.new))
2=> "/users"
3> app.polymorphic_path(RegistrationForm.new(User.last))
4=> "/users/1234"
For now, we have the same interface as the model.
Load Attributes
Since we can let form helper work correctly but we still cannot load the data when we edit an existing model.
To resolve it, we can adjust our initialize method to retrieve the necessary fields.
1class RegistrationForm < BaseForm
2 def initialize(record, params = {})
3 attributes = record.slice(:email, :password).merge(params)
4 super record, params
5 end
6end
Another way is to use Attribute API to support it, but we have to exactly define each attribute in our form object.
1class BaseForm
2 # ...
3 include ActiveModel::Attributes
4
5 def initialize(record, params = {})
6 @record = record
7 attributes = record.attributes.slice(*.self.class.attribute_names)
8 super attributes.merge(params)
9 end
10end
11
12# app/forms/registration_form.rb
13class RegistrationForm < BaseForm
14 attribute :email, :string
15end
But we have to take care the
params
hash, the model return attributes is{"name" => "Joy"}
but we use{name: "Joy"}
we will get the hash mixed string and symbol keys{"name" => "Joy", name: "Joy"}
and didn’t set the attribute to our form object.
Future Improve
In the current version, we have to pass the model instance to the form object. Maybe we can add some DSL to auto-create it.
1# Option 1
2class RegistrationForm < BaseForm
3 model_class 'User'
4
5 attribute :name
6end
7
8# Option 2
9class RegistrationForm < BaseForm[User]
10 attribute :name
11end
But we also need to consider this way may not a good idea in some complex system.
For example, we had load the User
from a controller or other object. But we cannot pass it to the form object. That means our form object usually loads the object when we access it.
If we have nested form it will cause the N+1 query in this case.
This is another topic when we use the form object or service object to refactor our code. We may reduce the duplicate code but cause our system to slow down or new hidden bug we didn’t know about it.
Conclusion
I didn’t have many experiences to use the form object. But I think it is a common case when we build an application. This version form object still has a lot of limitation and I didn’t consider all possible use cases.
I will try to improve it in my future works and keep it as a simple object if possible. I believe we didn’t always need to build a complex behavior and add a gem to resolve some simple things.